Timsort is an advanced sorting algorithm that efficiently sorts data by combining the strengths of two popular algorithms: merge sort and insertion sort. Developed by Tim Peters in 2002 for the Python programming language, Timsort is now the default sorting algorithm in Python and Java (for non-primitive types). It’s particularly well-suited for real-world data, especially when working with partially ordered datasets or data that exhibits specific patterns.
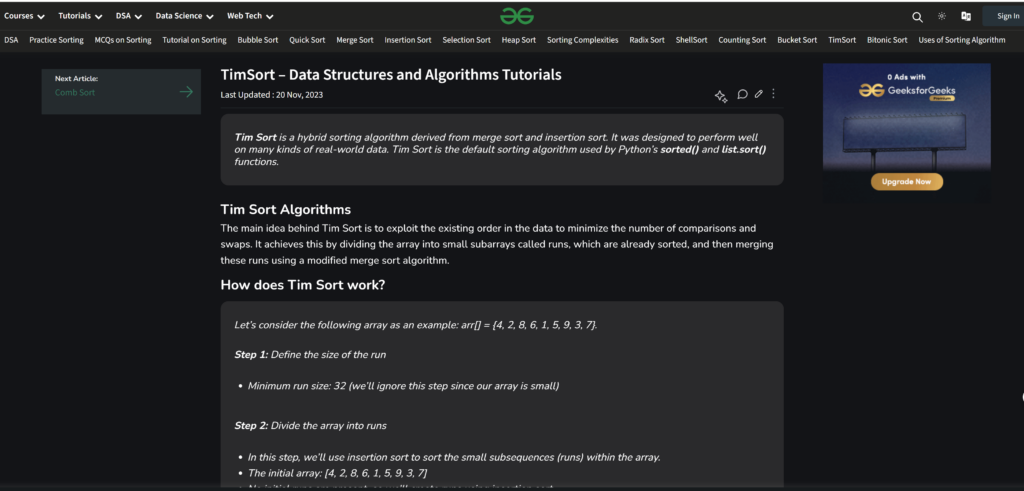
Key Features of Timsort
- Adaptive to Data: Timsort is highly efficient when sorting partially sorted datasets because it takes advantage of existing order within the data.
- Stable Sorting: As a stable sorting algorithm, Timsort preserves the relative order of elements with equal values. This is important when sorting complex data structures where maintaining the original order of equal elements matters.
- Efficient Time Complexity: With a time complexity of O(n log n) in the worst-case and average scenarios, Timsort is fast and efficient, even for large datasets.
- Hybrid Sorting Approach: Timsort combines merge sort (ideal for large datasets) and insertion sort (efficient for smaller datasets or subarrays), creating a powerful hybrid sorting method that adapts to the data it’s given.
How Timsort Works: A Simple Breakdown
Timsort is based on the concept of dividing the data into smaller chunks, sorting those chunks, and then merging them efficiently. Here’s how it works:
- Divide the Data into Runs: Timsort splits the data into small chunks called runs. These runs are sections of the dataset that are either already sorted or can be sorted quickly using insertion sort.
- Optimize the Runs: If a run is too small, Timsort extends it using insertion sort to meet a minimum run length, ensuring better overall performance.
- Merge the Runs: The sorted runs are then merged together using a merge sort-like approach, ensuring the final result is sorted efficiently and in a stable manner.
Why is Timsort So Popular?
Timsort is widely adopted in programming for several reasons:
- Optimized for Real-World Data: Timsort shines when sorting real-world datasets, which are often partially ordered or contain patterns that make sorting easier. This adaptability makes Timsort a go-to choice for many practical applications.
- Versatility Across Datasets: Whether you’re working with small or large datasets, Timsort provides excellent performance, making it a versatile algorithm for different use cases.
- Adoption in Major Programming Languages: The fact that Timsort is the default sorting algorithm in popular programming languages like Python and Java further proves its effectiveness. It’s trusted for everything from everyday programming tasks to complex data processing.
Benefits of Timsort for Developers
- Real-Time Performance: Timsort adapts dynamically, making it faster than other algorithms when dealing with datasets that are already partially sorted.
- Stability: The ability to maintain the order of equal elements means that Timsort is perfect for scenarios where you’re sorting complex structures, like records or objects, without disturbing the relative positions of items that are already equal.
- Efficient Memory Usage: Compared to other sorting algorithms, Timsort is known for being memory efficient while still offering top-tier performance.
Conclusion: Why Timsort is a Go-To Sorting Algorithm
In summary, Timsort is an efficient, adaptive sorting algorithm that excels at handling a wide variety of datasets. Its hybrid nature—combining the strengths of merge sort and insertion sort—makes it highly effective in real-world applications. Whether you’re working with large datasets or smaller, partially sorted ones, Timsort delivers consistent, reliable results.
Its stability, adaptability, and efficiency have made it the default sorting algorithm in major programming languages like Python and Java. If you’re working with data and need a reliable, fast sorting solution, Timsort is a great choice.